I’m honored to be able to post in Matt Groves‘ Annual C# Advent again this year, and today… December 22nd, 2018, is my second year contributing to it.
Last year I talked about ways to unload the main UI thread in WPF/.NET apps.
This year, I want to call attention to the Uno Platform tools I’ve been evangelizing for the past six months or so.
Silverlight is dead – Long Live Uno Platform!
To understand this perspective, we’ll need to walk through some key terms….
What is Silverlight?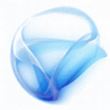
For those who don’t know, about ten years ago, Silverlight was the way to write C# and XAML to run in the web browser. It required a plug-in to run, much like Adobe Flash Player. Unfortunately, Microsoft announced the…. untimely demise of Silverlight in 2012. Silverlight, to some extent, seemed a more catchy term than other related technology names, so Microsoft used Silverlight as the name for mobile platforms that are also now depricated. As a result, it became almost synonymous with XAML.
What is XAML?
XAML, “eXtensible Application Markup Language” is the markup language behind a few great UI / UX layers in various Microsoft .NET-oid languages. For those who’ve used it, it’s an addictively cool language family. Using Visual Studio, Blend, and Adobe DX, you can create first-class UI. With features like Storyboard animation, basic animation becomes child’s play. Composition makes fast, dynamic animations easy. Once you’ve gotten the basic idea of it, one finds themselves wanting to use it anywhere they can… or at least that’s been my experience through WPF, Silverlight, Silverlight for Windows Phone, Silverlight for Windows Phone 8 / 8.1, Universal Windows Platform (UWP) and probably others.
The “code behind” XAML is typically C#, and historically .NET based.
What is Universal Windows Platform (UWP)?
UWP is the native platform of Windows 10. It’s similar to classic .NET in a few ways. First, UWP feels a lot like Windows Presentation Foundation (WPF) and .NET, being XAML and C# based, respectively. It differs from classic .NET because it has a lot of fixes, both in terms of security and performance, that .NET can’t afford to apply for various reasons. More simply put, .NET had some serious technical debt built up, so the easiest way to forgive that debt was to build a new platform based on the old languages. Your XAML and C# skills are the same, but the namespaces and supporting framework libraries are different.
Don’t fret, though… UWP runs natively on over 800 million devices (as of today, December 22nd, 2018), and that number continues to grow. UWP is the native platform for all Windows 10 devices. This means desktops, laptops, tablets, phones, HoloLenses, Xbox consoles, IoT embedded devices, and more.
What is WebAssembly?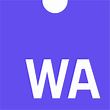
WebAssembly is a relatively new bytecode language specification… a virtual machine specification, similar to the Java Virtual Machine (JVM), that is fully supported by most modern major web browsers. It allows near native performance in the same sandbox that javascript apps run in. When you run javascript in a web page, the jit compiler in the browser converts the code into tokenized bytecode in order to execute it quicker. WebAssembly improves on this significantly by pre-compiling the code. Because the code is pre-complied, it doesn’t have to be sourced from javascript. It can be compiled from just about any programming language. Wasm, as it’s called, went from a specification just a few short years ago to being well supported in all major modern web browsers.
What is Uno Platform?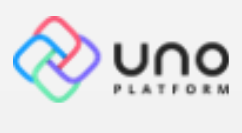
Uno Platform, for our purposes, is not really a new platform, but an extension to UWP.
You write your UWP application for your Windows 10 devices the same way you always have. Uno provides a mechanism to re-compile that UWP app to Web Assembly (and… by the way… using Xamarin tools, also to iOS… and also to Android!)
In a sense, Uno Platform is to UWP as Xamarin is (roughly) to classic .NET.
See the connection?
Let’s do some math…
UWP = C# & XAML for Windows 10. (800,000,000 devices)
Uno Platform += UWP for iOS (Millions more devices), Android (over a Billion devices), and WebAssembly (every modern major PC in the world)
Now factor in this…
.NET Core 3 += UWP for services
What does all that add up to?
One skill set…
UWP (C# & XAML) = FULL STACK, on all major platforms
From data access layer to REST API to UI canvas.
Wait a minute… What about Xamarin?
Xamarin is the older way to do C# for cross platform / mobile.
Coincidentally, just this past Thursday, Carl Barton, a Microsoft MVP for Xamarin presented the Xamarin Forms Challenge at the Windows Platform App Devs users group. The goal of the meetup was to demonstrate creating a simple app in C# and running it on as many platforms as we could in the hour. He easily pushed ran the app on over a dozen platforms in the hour.
Uno Platform actually depends on Xamarin libraries to support iOS and Android.
The main differences between Xamarin and Uno Platform are these:
- Xamarin encourages you to use a Xamarin-specific dialect of XAML, including Xamarin Forms to express your cross platform UI.
- If you already know & understand Microsoft’s UWP dialect of XAML, Uno Platform uses that dialect.
- Xamarin enables you to produce binaries for dozens of different target platforms, reaching a billion or more devices. These include .NET, UWP, iOS, Android, Tizen, Unity, ASP.NET, and many others.
- Uno Platform only enables you to reach three additional binary output targets… iOS, Android, and WebAssembly…. but WebAssembly can or likely will soon cover most of what Xamarin Forms covers.
I’ll leave it up to you which to choose, but for me, given the choice between Xamarin with several years of technical debt built up in a distinct dialect of XAML, and Uno Platform, using the fresher, native UWP dialect of XAML…
Finally…
Here’s the slides I presented most recently at the New England Microsoft Developers meetup in Burlington, Mass on December 6th (thanks again to Mathieu Filion of nventive for much of the content):
[office src=”https://onedrive.live.com/embed?cid=90A564D76FC99F8F&resid=90A564D76FC99F8F%211297452&authkey=AM6QZrb_6-9ltdE&em=2″ width=”402″ height=”327″]